This module consist of a DHT11 digital humidity and temperature sensor and a 1 kΩ resistor. The DHT11 uses an internal thermistor and a capacitive humidity sensor to determine environment conditions, an internal chip is responsible for converting readings to a serial digital signal.
Humidity
Humidity is the amount of water vapor in the air. Water vapor is the gaseous state of water and is invisible. Humidity indicates the likelihood of precipitation, dew, or fog. Higher humidity reduces the effectiveness of sweating in cooling the body by reducing the rate of evaporation of moisture from the skin.
DHT11 Humidity Temperature Sensor
The DHT11 humidity and temperature sensor measures relative humidity (RH) and temperature. Relative humidity is the ratio of water vapor in air vs. the saturation point of water vapor in air. The saturation point of water vapor in air changes with temperature. Cold air can hold less water vapor before it is saturated, and hot air can hold more water vapor before it is saturated. The formula for relative humidity is as follows:
Relative Humidity = (density of water vapor / density of water vapor at saturation) x 100%
Basically, relative humidity is the amount of water in the air compared to the amount of water that air can hold before condensation occurs. It’s expressed as a percentage. For example, at 100% RH condensation (or rain) occurs, and at 0% RH, the air is completely dry.
Specifications
- Supply voltage: 3.3 ~ 5.5V DC
- Output: single-bus digital signal
- Measuring range: Humidity 20-90% RH, Temperature 0 ~ 50 ℃
- Accuracy: Humidity + -5% RH, temperature + -2 ℃
- Resolution: Humidity 1% RH, temperature 1 ℃
- Long-term stability: <± 1% RH / Year
Arduino Connection with KY-015 Temperature and Humidity Sensor
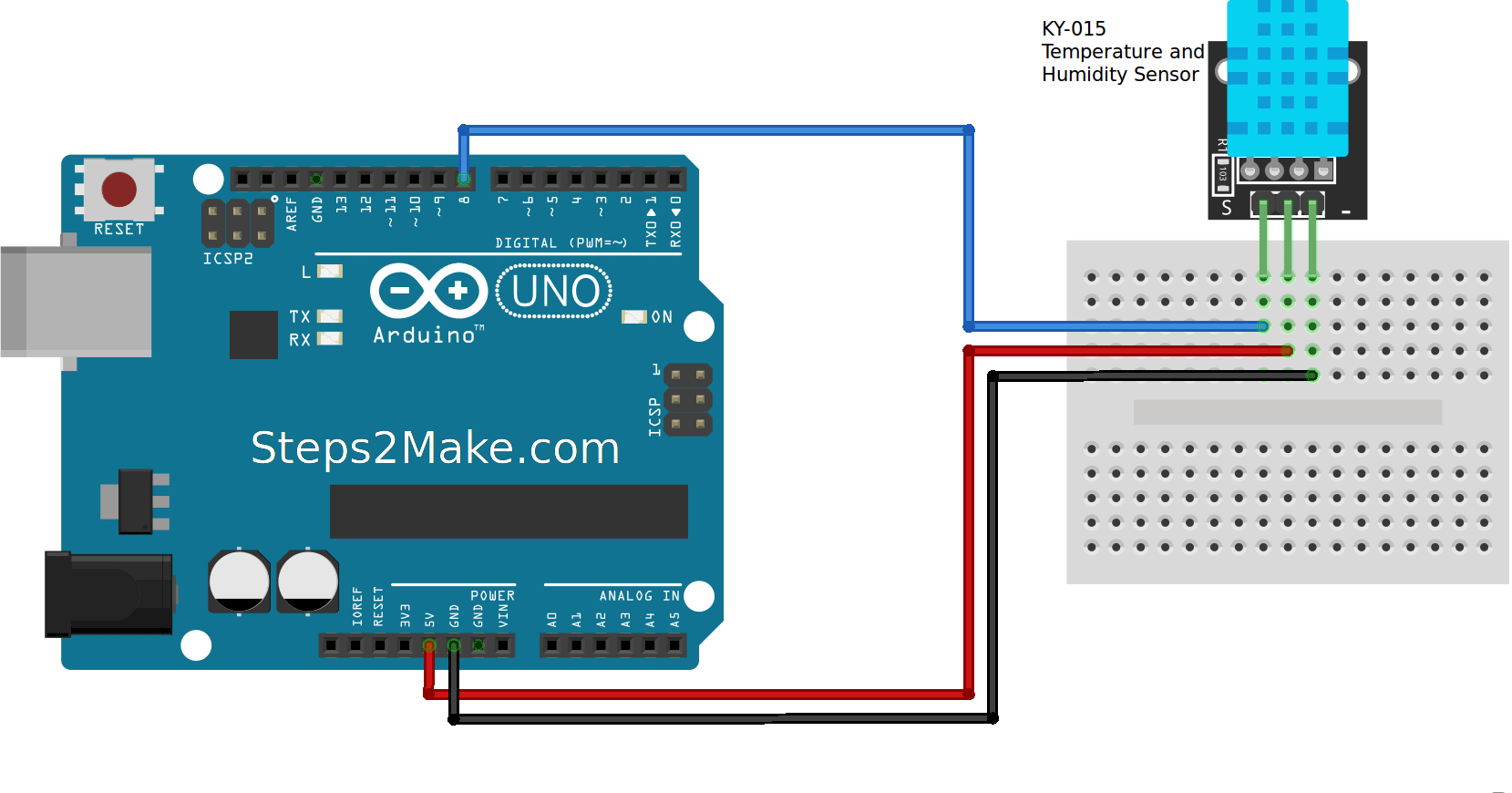
Arduino pin 8 –> Pin S module
Arduino GND –> Pin – module
Arduino +5 –> Pin Middle
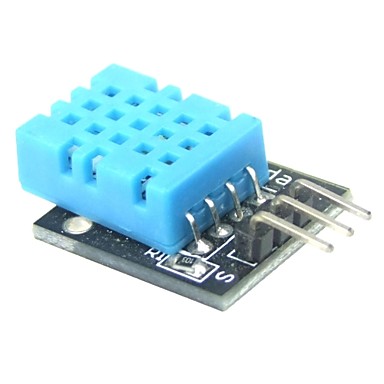
Arduino Program for KY-015 Temperature and Humidity Sensor
For this program DHT Library is required download from here https://github.com/adafruit/DHT-sensor-library
//Humidity and Temperature Measurement //steps2make.com #include <dht.h> dht DHT; #define DHT11_PIN 8 void setup(){ Serial.begin(9600); } void loop() { int chk = DHT.read11(DHT11_PIN); Serial.print("Temperature = "); Serial.println(DHT.temperature); Serial.print("Humidity = "); Serial.println(DHT.humidity); delay(1000); }
If you want to program it without library use this program
//KY015 DHT11 Temperature and humidity sensor int DHpin = 8; byte dat [5]; byte read_data () { byte data; for (int i = 0; i < 8; i ++) { if (digitalRead (DHpin) == LOW) { while (digitalRead (DHpin) == LOW); // wait for 50us delayMicroseconds (30); // determine the duration of the high level to determine the data is '0 'or '1' if (digitalRead (DHpin) == HIGH) data |= (1 << (7-i)); // high front and low in the post while (digitalRead (DHpin) == HIGH); // data '1 ', wait for the next one receiver } } return data; } void start_test () { digitalWrite (DHpin, LOW); // bus down, send start signal delay (30); // delay greater than 18ms, so DHT11 start signal can be detected digitalWrite (DHpin, HIGH); delayMicroseconds (40); // Wait for DHT11 response pinMode (DHpin, INPUT); while (digitalRead (DHpin) == HIGH); delayMicroseconds (80); // DHT11 response, pulled the bus 80us if (digitalRead (DHpin) == LOW); delayMicroseconds (80); // DHT11 80us after the bus pulled to start sending data for (int i = 0; i < 4; i ++) // receive temperature and humidity data, the parity bit is not considered dat[i] = read_data (); pinMode (DHpin, OUTPUT); digitalWrite (DHpin, HIGH); // send data once after releasing the bus, wait for the host to open the next Start signal } void setup () { Serial.begin (9600); pinMode (DHpin, OUTPUT); } void loop () { start_test (); Serial.print ("Current humdity ="); Serial.print (dat [0], DEC); // display the humidity-bit integer; Serial.print ('.'); Serial.print (dat [1], DEC); // display the humidity decimal places; Serial.println ('%'); Serial.print ("Current temperature ="); Serial.print (dat [2], DEC); // display the temperature of integer bits; Serial.print ('.'); Serial.print (dat [3], DEC); // display the temperature of decimal places; Serial.println ('C'); delay (700); }
Results
Open serial monitor and observe the readings. Blowing air on sensor will change the humidity readings.
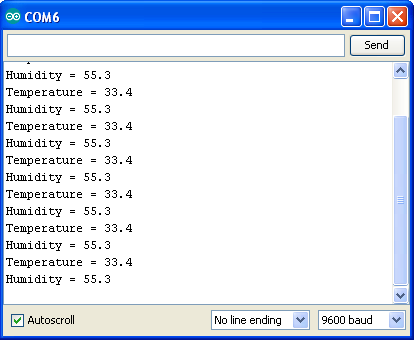
Responses